The simple CMyApp application below creates a standard window including a title, a system menu and the standard minimise, maximise and a close box. No data member functions are declared and just one virtual function, InitInstance, is overwritten.
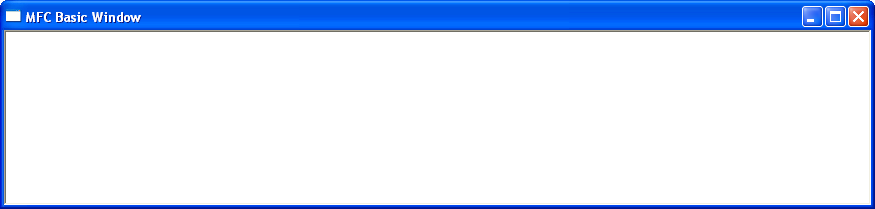
#include <afxwin.h> class CSimpleApp : public CWinApp { public: BOOL InitInstance(); }; class CMainFrame : public CFrameWnd { public: CMainFrame(); }; CSimpleApp MFCApp1; BOOL CSimpleApp::InitInstance() { m_pMainWnd = new CMainFrame(); m_pMainWnd->ShowWindow(m_nCmdShow); return TRUE; } CMainFrame::CMainFrame() { Create(NULL, TEXT("MFC Basic Window")); }
Line 1 – AFXWIN.H contains all the core and standard components and must be included in any MFC application.
Line 2 to 6 – To create the most basic application requires that a class must first be derived from the MFC’s CWinApp base class. CWinApp contains functions for initialising and running the application. The InitInstance member function is inherited from CWinApp and must be overridden by default. Returning true from InitInstance signals that application initialisation can proceed; returning FALSE shuts down the application. InitInstance is the place to perform any initialisations necessary each time the program starts
Line 8 to 12 – The basic functionality of a window is defined in a class called CWnd. The CWnd class gives birth to a derived class called CFrameWnd which describes a window and defines what a window looks like. The description of a window includes items such as its name, size, location, etc.
Line 14 – Instantiates a global application object. There must be only one object of type CWinApp for each application.
16 to 21 – The InitInstance member function creates a new CMainFrame object on the heap. A pointer to this new window object is stored in the public variable m_pMainWnd and it’s through this pointer that the application class maintains contact with its main window. After instantiating the window object, the window is displayed by calling the window object’s ShowWindow() function. ShowWindow accepts just one integer parameter that specifies whether the window should initially be shown minimized, maximized, or neither minimized nor maximized.
23 to 26 – The CMainFrame class constructor, creates the main window by executing the CFrameWnd member function Create. Create returns non-zero if it’s successful and zero if the window cannot be created.
Further information about the Create function can be found in the link below.
https://docs.microsoft.com/en-us/cpp/mfc/reference/cframewnd-class?view=vs-2019#create