The simple Windows program below creates a basic window with a system menu icon, a minimise, maximise and a close box. It can be compiled in either C or C++.
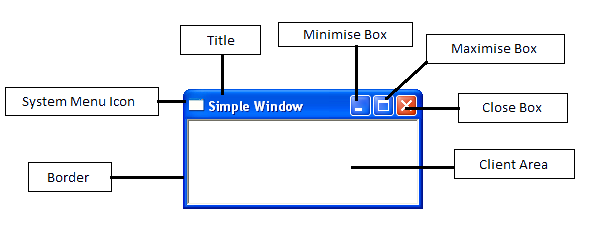
#include <windows.h> LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM); int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,LPSTR lpCmdLine, int nCmdShow) { WNDCLASSEX wc; MSG msg; //Registering the Window Class wc.cbSize = sizeof(WNDCLASSEX); wc.style = 0; wc.lpfnWndProc = WndProc; wc.cbClsExtra = 0; wc.cbWndExtra = 0; wc.hInstance = hInstance; wc.hIcon = LoadIcon(NULL, IDI_APPLICATION); wc.hCursor = LoadCursor(NULL, IDC_ARROW); wc.hbrBackground = (HBRUSH)(COLOR_WINDOW+1); wc.lpszMenuName = NULL; wc.lpszClassName = TEXT("myWindowClass"); wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION); RegisterClassEx(&wc); //Creating the Window CreateWindowEx(WS_EX_CLIENTEDGE,TEXT("myWindowClass"),TEXT("Simple Window"), WS_VISIBLE | WS_OVERLAPPEDWINDOW,CW_USEDEFAULT, CW_USEDEFAULT, 240, 120, NULL, NULL, hInstance, NULL); //The Message Loop while(GetMessage(&msg, NULL, 0, 0) > 0) { TranslateMessage(&msg); DispatchMessage(&msg); } return msg.wParam; } //WndProc procedure. Application acts on messages LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) { switch(msg) { case WM_CLOSE: DestroyWindow(hwnd); break; case WM_DESTROY: PostQuitMessage(0); break; default: return DefWindowProc(hwnd, msg, wParam, lParam); } return 0; }
Line 1 – All Windows programs must include the header file <windows.h>. This contains declarations for all of the functions in the Windows API, all the common macros used by Windows programmers, and all the data types used by the various functions and subsystems.
Line 2 – Function declaration for CALLBACK function WndProc().
Line 3 – WinMain() function. Marks the program entry point.
Line 5 – Declares the wc structure variable that defines the Window’s class.
Line 6 – Declares the msg structure variable for holding Windows messages.
Lines 9 to 20 – Defines the Window’s class
Line 21 – Registers the Windows class using the function RegisterClassEx().
Line 24 – Once a Window has been defined and registered it is created using the API function CreateWindowEx().
Lines 27 to 33 – The final part of WinMain is the message loop. The purpose of the message loop is to receive and process messages sent from Windows. Once the message loop terminates the value of msg.wParam is returned to Windows.
Line 36 to 50 – The WndProc() procedure is used by Windows to pass messages to an application. In this instance, only the WM_DESTROY & WM_CLOSE message is explicitly processed.