Basic Text Output
Two popular text printing functions are TextOut() and DrawText().
The prototypes are as follows –
BOOL TextOut(HDC hdc, int x,int y,LPCSTR lpString,int slength);
where
hdc – A handle to the device context.
x – horizontal location.
y – vertical location.
lpString – pointer to a character string.
slength – is the number of characters in the string to be displayed
If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
int DrawText( HDC hdc,LPCTSTR lpString,int nCount,LPRECT lpRect,UINT uFormat);
where
hdc – A handle to the device context.
lpString – A pointer to the string that specifies the text to be drawn. If the nCount parameter is -1, the string must be null-terminated.
nCount – The length, in characters, of the string
lpRect – A pointer to a RECT structure containing the rectangle (in logical coordinates) in which the text is formatted.
uFormat – The method of formatting the text.
If the function succeeds, the return value is the height of the text in logical units. If the function fails, the return value is zero
Creating Custom Fonts
In addition to a small variety of built-in fonts, Windows also allows the creation of custom-built fonts. To create a custom font use the CreateFont() win32 API function. The syntax for the CreateFont function is
HFONT CreateFont(int cHeight,int cWidth,int cEscapement,int cOrientation,int cWeight,DWORD bItalic,DWORD bUnderline,DWORD bStrikeOut,DWORD iCharSet,DWORD iOutPrecision,DWORD iClipPrecision,DWORD iQuality,DWORD iPitchAndFamily,LPCSTR pszFaceName);
If successful the CreateFont returns a handle to the font created. If the function fails, the return value is NULL. Any created font must be deleted before the application terminates. In order to delete the font use the DeleteObject() function.
For a more detailed explanation of font creation – https://docs.microsoft.com/en-us/windows/win32/gdi/font-creation-and-selection
Before any graphics object can be used it is ‘selected’ into the current device context (DC). The new object will then replace the previous graphic object of the same type.
Setting Text Background and Text Colour
The Windows API functions SetTextColor() and SetBkColorTo() are used to set the text and text background colour. The syntax for these two are
COLORREF SetTextColor(HDC hdc,COLORREF color);
COLORREF SetBkColor(HDC hdc,COLORREF color);
Where hdc refers to the device context and colour is used to specify an RGB value. If the function succeeds, the return value is a colour reference to the previous text colour.
TextMetrics
The textmetric structure describes the attributes of a given font and enables an application to work with text with different font attributes. The API function GetTextMetrics() is used to get information about the current font. The syntax of this function is –
BOOL GetTextMetrics(HDC hdc,LPTEXTMETRIC lptm);
Where hdc is a handle to the device context and lptm is a pointer to the TEXTMETRIC structure that receives the text metrics.
If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
The textmetric structure is defined as follows –
TypeDef struct TEXTMETRIC{
tmHeight As Long //height of font
tmAscent As Long //height above baseline
tmDescent As Long //length of descender
tmInternalLeading As Long //space above character
tmExternalLeading As Long //blank space above rows
tmAveCharWidth As Long //average width
tmMaxCharWidth As Long //maximum width
tmWeight As Long //weight
tmOverhang As Long //extra width added to special font
tmDigitizedAspectX As Long //horizontal aspect
tmDigitizedAspectY As Long //vertical aspect
tmFirstChar As Byte //first character in font
tmLastChar As Byte //last character in font
tmDefaultChar As Byte //dafault character
tmBreakChar As Byte //character used to break words
tmItalic As Byte //non zero if italic
tmUnderlined As Byte //non zero if underlined
tmStruckOut As Byte //non zeri is struckout
tmPitchAndFamily As Byte //pitch and family of font
tmCharSet As Byte //character set identifier
}End Type
For more information
https://docs.microsoft.com/en-gb/windows/win32/api/wingdi/ns-wingdi-textmetrica
Character Spacing and Creating Multi-line Text
Since the characters in a non-monospaced typeface will not occupy the same amount of horizontal space on a line, it will be necessary for an application to know the length of a string when outputting consecutive lines of text. As Windows does not keep track of the current output location an application will need to know where any previous text output ended. To provide this information the Windows API includes the function GetTextExtentPoint32(). The syntax of this function is
BOOL GetTextExtentPoint32(HDC hdc,LPCSTR lpString,int len,LPSIZE lpsize);
where
hdc – A handle to the device context.
lpString – holds the string used for the length calculation.
len – length of the string lpString.
lpsize – A pointer to a SIZE structure that returns the width or height of the string (see below)
If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
The prototype for the size structure is
typedef struct tagSIZE {LONG cx;LONG cy;} SIZE;
Upon return, the CX field will contain the length of the string and the CY will contain the height of the string.
Example
The following short program demonstrates various stock and user-defined fonts and other aspects of font formatting
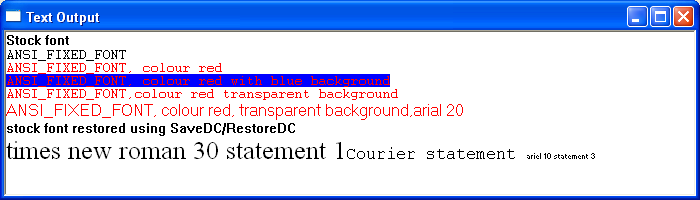