A dialog box is a temporary popup window that prompts the user for additional information. Dialog boxes are usually created using using dialog editor and defined within a programs resource file. A dialog box will usually contain one or more controls (child windows) with which the user can enter text, choose options, or direct the action of the application.
In addition to user-defined dialogue boxes, Windows also provides predefined dialog boxes that support common menu items such as colour selection and an open file interface.
Modal v Modeless Dialogue Box
Dialog boxes can be either modeless or modal. A modal dialogue box will not allow the user to switch between the dialog box and any other window without explicitly closing the dialog box. A modeless dialog box does not prevent the user from switching to other parts of the application.
Creating a Modeless Dialog Box
Modeless dialog boxes are created using the API function CreateDialog(). The syntax is below
hDlgModeless = CreateDialog (hInstance, lpTemplate, hWndParent, lpDialogFunc) ;
hInstance – A handle to the current application.
lpTemplate – specifies the resource identifier of the dialog box template.
hWndParent – A handle to the parent window that owns the dialog box.
lpDialogFunc – A pointer to the dialog box procedure.
Return value – If the function succeeds, the return value is the dialog box handle. If the function fails, the return value is NULL.
Deactivating a Modeless Dialog Box
To destroy the modeless dialog box, use the DestroyWindow() function.
BOOL DestroyWindow(HWND hWnd);
Where HWnd is a handle to the window to be destroyed. If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
Creating a Modal Dialog Box
Modal dialog boxes are created using the APO function DialogBox(). The syntax is
void DialogBox(hInstance,lpTemplate,hWndParent,lpDialogFunc);
hInstance – A handle to the current application.
lpTemplate – Specifies the resource identifier of the dialog box template.
hWndParent – A handle to the parent window that owns the dialog box.
lpDialogFunc – A pointer to the dialog box procedure.
Deactivating a Modal Dialog Box
To deactivate and close a modal dialogue box use the EndDialog() API function call. The syntax of this function is
BOOL EndDialog(HWND hDlg,INT_PTR nResult);
HDlg – A handle to the dialog box to be destroyed.
nResult – The value to be returned to the application from the function that created the dialog box.
Return value – If the function succeeds, the return value is nonzero. If the function fails, the return value is zero.
Processing Dialog Messages
Each dialogue box will have its version of the window call-back function (wndproc) and receive messages in the same fashion as the main window. The dialog callback function, however, differs from its main Windows counterpart in that it returns a boolean value.
Example
The code example demonstrates a modal and modeless dialog box. The modal dialog box displays a simple message. The modeless dialog box has 3 radio buttons. Selecting any of the radiobutton changes the background colour of the parent window.
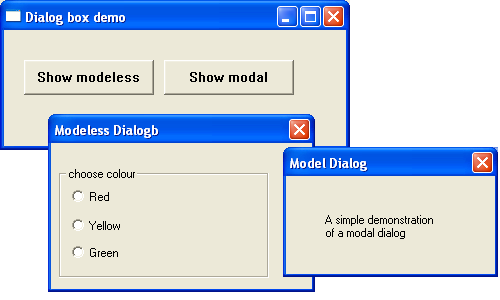
Dialog-Based Applications
Creating a program where the main window is a dialog box is referred to as a dialog-based application. This method simplifies the application build process by making it easier to place controls onto the main window using the system resource dialog editor. A dialog box procedure can then be added to react to events that occur in the dialog box. Dialog-based applications are much simpler, architecturally, than other designs therefore dialog applications are best suited for creating rudimentary apps.
The following example creates a simple dialog-based application. The dialog box title can be changed to the value in the textbox by clicking the button control.
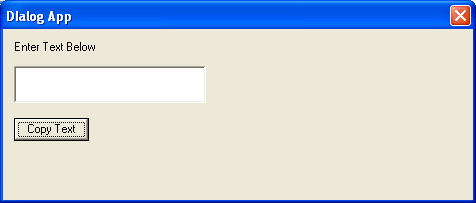