A child window is a window that exists inside and is dependent on a parent window. The child window processes mouse and keyboard messages and notifies the parent window when the child window’s state has changed. A standard control is created by instantiating one of the MFC control classes and calling the associated object’s create member function.
Windows makes the classic controls available to the application programs it hosts by registering six predefined WNDCLASS’s. The control types, their WNDCLASS’s, and the corresponding MFC classes are shown in the following table.
Control Type | WNDCLASS | MFC Class |
---|---|---|
Buttons | “BUTTON” | CButton |
List boxes | “LISTBOX” | CListBox |
Edit controls | “EDIT” | CEdit |
Combo boxes | “COMBOBOX” | CComboBox |
Scroll bars | “SCROLLBAR” | CScrollBar |
Static controls | “STATIC” | Cstatic |
Buttons
MFC’s CButton class encapsulates the Windows button controls. A Button is a simple control used to trigger an action. The appearance and behaviour of a button control are specified when it is created by including the appropriate style flag in the button’s window style. A button can have many different appearances such as a push-button, radio button, or checkbox.
Checkbox
A checkbox is a small square box with an associated descriptive label. Checkboxes function as a toggle switch switch between selected and de-selected. Clicking the box once causes a checkmark to appear; clicking again toggles the checkmark off. Checkboxes can be created with the following style
BS_CHECKBOX – Creates a small, empty check box with text. By default, the text is displayed to the right of the check box.
BS_AUTOCHECKBOX – Creates a button that is the same as a check box, except that the check state automatically toggles between checked and cleared each time the user selects the check box.
BS_3STATE – Creates a button that is the same as a check box, except that the box can be greyed in addition to being checked or cleared.
BS_AUTO3STATE – Creates a button that is the same as a three-state check box, except the box changes its state when the user selects it. The checkbox state cycles through checked, indeterminate, and cleared.
The CEdit member functions GetState() and GetState() can be used to check/set the state of a button and ON_BN_CLICKED macro maps the button click function to the button click event.
For a further reading on the CButton class
https://docs.microsoft.com/en-us/cpp/mfc/reference/cbutton-class?view=vs-2019
The following short program uses 3 checkboxes to change the window’s background colour. Selecting combinations of each produces a mixture of red green and blue. The initial default background colour is black while selecting all 3 buttons will produce white.
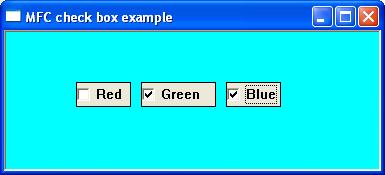
Download Code
RadioButton
A radio button is a small circular box with an associated descriptive label. Radio buttons normally come in groups that allow the user to choose only one of a predefined set of mutually exclusive options. Radiobuttons can be created with one of the following styles
BS_RADIOBUTTON – checking and unchecking are done manually
BS_AUTORADIOBUTTON – checking and unchecking are done automatically. When clicked, a radio button checks itself and unchecks the other radio buttons in the group.
For a further reading on the CButton class
https://docs.microsoft.com/en-us/cpp/mfc/reference/cbutton-class?view=vs-2019
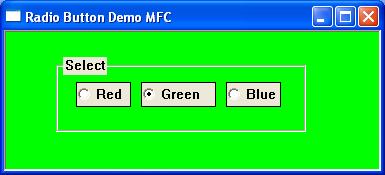
Download Code
Static control
MFC’s CStatic class encapsulates the Windows static control. Static controls are commonly used as labels for other controls. A static control displays text, shapes and pictures such as icons or bitmaps. The static control cannot be selected, accept input from the keyboard or mouse, and does not send WM_COMMAND messages back to the parent window.
For further reading on the CStatic classes
https://docs.microsoft.com/en-us/cpp/mfc/reference/cstatic-class?view=vs-2019
Editbox
MFC’s CEdit class encapsulates the functionality of edit controls. Edit controls are used for text entry and editing and come in two varieties: single-line and multiline,
For further reading on the CEdit class
https://docs.microsoft.com/en-us/cpp/mfc/reference/cedit-class?view=vs-2019
In the following example clicking the button title ‘set button’ changes the contents of the static box to the value of the textbox. The MFC CEdit function GetWindowTextW() and the MFC SetWindowTextW() are used to get and set the contents of both the editbox and staticbox.
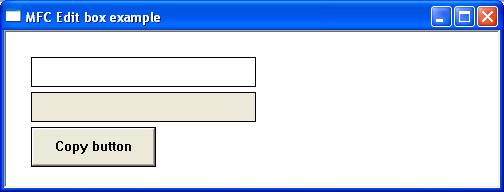
Download Code
ScrollBar
MFC’s CScrollBar class encapsulates scroll bar controls. A scroll bar is an object that allows the user to adjust a particular value, a section of the window or view, by navigating either left and right or up and down. A scroll bar appears as a long bar with a small button at each end. Between these buttons, there is a moveable bar called a thumb. Scrollbars exist in two forms: the standard scroll bar and the scroll bar control. The standard scroll bar is an integral part of a window, whereas the scroll bar control exists as a separate control
The most common scrollbar messages are
SB_LINEUP – is sent when the scrollbar moves up one position
SB_LINEDOWN – is sent when the scrollbar moves down one position
SB_PAGEUP – is sent when the scrollbar is moved up one page
SB_PAGEDOWN – is sent when the scrollbar is moved down one page
SB_LINELEFT – is sent when the scrollbar is moved left one position
SB_LINERIGHT – is sent when the scrollbar is moved right one position
SB_PAGELEFT – is sent when the scrollbar is moved one page left
SB_PAGERIGHT – is sent when the scrollbar is moved one page right
SB_THUMBPOSITION – is sent after the thumb bar is dragged to a new position
SB_THUMBTRACK – is sent while the thumb bar is dragged to a new position
The CScrollbar member function SetScrollRange() is used to set the minimum and maximum scroll box positions and the GetScrollPos() and SetScrollPos() member functions retrieve/set the current position of the scroll box (thumb).
Scrollbar information is passed to the parent windows by use of the ON_WM_VSCROLL and ON_WM_HSCROLL message macros
For further reading on the CScrollBar class
https://docs.microsoft.com/en-us/cpp/mfc/reference/cscrollbar-class?view=vs-2019
The following short program demonstrates a horizontal scrollbar control. The scrollbar position is shown in the static class.
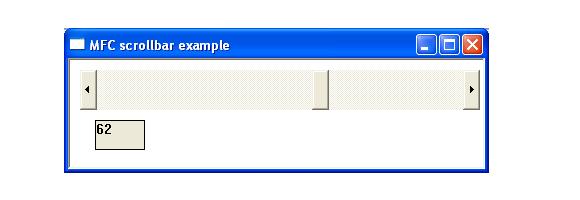
Listbox
MFC’s Clistbox Class encapsulates the Windows listbox control. A listbox displays a list of selectable items in a scrollable box. Users can select or deselect one or more of these items by clicking the appropriate line of text. For further reading on the CListBox class
https://docs.microsoft.com/en-us/cpp/mfc/reference/clistbox-class?view=vs-2019
The following short program creates a listbox and then adds a limited number of selectable items. Clicking any list item results in the selected listview value being copied to the static box. Clicking the ad button adds a new record, clicking the amend button amends the selected listview value, and clicking the delete button deletes the selected listview item. The Clist member functions AddString(), DeleteString() and GetString() are used to set the add, delete and get the selected listbox content and the ON_LBN_SELCHANGE maps the combo box change event to the associated function.
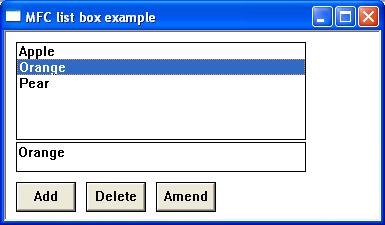
Download Code
Combo Box
MFC’s CComboBox class encapsulates the functionality of the ComboBox controls. A combo box or drop-down list is a combination of a listbox and editbox, allowing the user to either type a value directly or select a value from the list. The following list outlines possible combo-box styles.
For further reading on the CComboBox class
https://docs.microsoft.com/en-us/cpp/mfc/reference/ccombobox-class?view=vs-2019
The following short program displays a dropdown list or combo box. Items can be added deleted or amended using the textbox and the appropriate button.
The CComboBox member functions AddString(), DeleteString(), and GetLBText() are used to set the add, delete and get the selected listbox content. The ON_LBN_SELCHANGE maps the list box change event to the associated function.
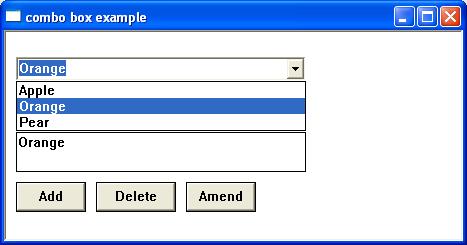
Download Code