Displaying Text
The two most popular functions for displaying text are the CDC member functions TextOut() and DrawText(). All CDC text functions use the font that is currently selected into the device context The syntax for these two member functions are –
virtual BOOL TextOut(int x,int y,LPCTSTR lpszString,int nCount);
BOOL TextOut(int x,int y,const CString& str);
Where
x – Specifies the vertical position of the starting point of the text.
y – Specifies the horizontal position of the starting point of the text.
lpszString – Points to the character string to be drawn.
nCount – Specifies the number of characters in the string.
str – A CString object that contains the characters to be drawn.
Returns non-zero if the function is successful; otherwise zero.
virtual int DrawText(LPCTSTR lpszString,int nCount,LPRECT lpRect,UINT nFormat);
int DrawText(const CString& str, LPRECT lpRect,UINT nFormat);
where
pszString – Points to the string to be drawn.
nCount – Specifies the number of chars in the string.
lpRect – Points to a RECT structure or CRect object that contains the text to be formatted.
str – A CString object that contains the specified characters to be drawn.
nFormat – Specifies the method of formatting the text.
The function returns the height of the text if the function is successful.
Fonts
In MFC, the CFont class is used to create and manipulate fonts. By default, the CDC text output class can draw text using a pre-selected system. MFC supports 7 built-in fonts. These are
ANSI_FIXED_FONT
ANSI_VAR_FONT
DEVICE_DEFAULT_FONT
DEFAULT_GUI FONT
OEM_FIXED_FONT
SYSTEM_FONT
SYSTEM_FIXED_FONT
To select one of these stock fonts into the current device context first create a CFont object and then call the object member function CreateStockObject() using one of the custom font names above. The CFont object can then be selected into the current device context by calling the SelectObject() member function as below –
CFont newfont;
Newfont.CreateStockObject(ANSI_FIXED_FONT);
paintDC.SelectObject(newfont);
Custom Fonts
The CFont object class supplies 4 member functions for creating and initialising a font before use: CreateFont(), CreateFontIndirect(), CreatePointFont(), and CreatePointFontIndirect(). Use CreateFont or CreateFontIndirect to specify the font size in pixels, and CreatePointFont and CreatePointFontIndirect to specify the font size in points. The syntax for the CreateFont member function is
BOOL CreateFont( int nHeight, int nWidth,int nEscapement, int nOrientation,int nWeight,BYTE bItalic, BYTE bUnderline,BYTE bStrikeOut,BYTE nCharSet,BYTE nOutPrecision,BYTE nClipPrecision, BYTE nQuality,BYTE nPitchAndFamily, LPCTSTR lpszFacename);
where
nHeight – The height, in logical units, of the font.
nWidth – The average, in logical units, of the font.
nEscapement – Angle of the escapement.
nOrientation – Base-line orientation angle
nWeight – Font weight ( 0 – 1000)
bItalic – Specifies an italic font.
bUnderline – Specifies an underlined font.
bStrikeOut – A strikeout font if set to TRUE.
nCharSet – Character set identifier
nOutPrecision – Defines how closely the output must match the requested font attributes
nClipPrecision – Defines how to clip characters partially outside the clipping region.
nQuality – Defines how carefully GDI must attempt to match the logical font attributes to those of an actual physical font.
nPitchAndFamily -The two low-order bits specify the pitch of the font
pszFaceName – Is a pointer to a null-terminated string that specifies the typeface name of the font
If the function succeeds, the return value is a handle to a logical font. If the function fails, the return value is NULL.
For further detailed reading of the CreateFont member function
https://docs.microsoft.com/en-us/cpp/mfc/reference/cfont-class?view=vs-2019#createfont
For further detailed reading on the CFont MFC class
https://docs.microsoft.com/en-us/cpp/mfc/reference/cfont-class?view=vs-2019
Deleting GDI Objects
Fonts like other objects created from GDI Object classes are resources that consume space in memory. It is therefore important that they are deleted when no longer required. If a CFont object is created on the stack it will automatically be deleted when it goes out of scope. If a CFont object is created on the heap it must be explicitly deleted by a call to the object member function DeleteObject(). Stock objects, even if they are “created” with CreateStockObject never need to be deleted. Failure to delete GDI objects leads to a “memory leak” and gradual depletion of available system memory.
Setting Text Colour.
The cdc member function SetTextColor() sets the text colour to the specified colour. The syntax for this function is –
settextcolor(colorref color);
Where color specifies the colour of the text as an RGB colour value.
Returns an RGB value for the previous text colour.
Setting the Text Background Colour
The cdc member function SetTextColor sets the text background to the specified colour. The syntax for this function is
virtual COLORREF SetBkColor(COLORREF colour);
Where colour specifies the new background colour.
Returns the previous background colour as an RGB colour value. If an error occurs, the return value is 0x80000000.
Setting the Text Background Display Mode
The background mode defines whether the system removes existing background colours before drawing text. To set the way text is displayed against its background use the cdc function SetBkMode(). The device context text background can be set to opaque or transparent. The prototype for this function is
SetBkMode(int nBkMode)
where
nBKMode – Specifies the mode to be set. This parameter can be either of the following values:
- opaque – Background is filled with the current background colour before the text, hatched brush, or pen is drawn. This is the default background mode.
- transparent – Background is not changed before drawing.
Returns the previous background mode.
Textmetric
The textmetric structure describes the attributes of a given font and enables an application to work with text with different font attributes. Knowing the structure of a particular font is necessary because Windows only provides minimal support for text processing. Attributes such as character height and distance between lines must be factored into any application supporting text output. The MFC member function used to obtain the text metric of the existing font is the CDC member function GetTextMetrics(). The syntax for this function is
BOOL GetTextMetrics(LPTEXTMETRIC lpMetrics) const;
Where lpMetrics points to the TEXTMETRIC structure that receives the metrics.
For detailed reading on the textmetric structure use the following resource
https://docs.microsoft.com/en-us/windows/win32/api/wingdi/ns-wingdi-textmetricw
Character Spacing
For an application to output consecutive lines of text, the length of a string will need to be known so that any following lines of text will appear in the correct location. This is necessary because Windows does not keep track of the current text output location. Since the length of a string will not be consistent in a non-monospaced typeface, MFC includes the cdc member function GetTextEntent(). This function enables an application to keep track of the horizontal space taken up by the currently selected device context font.
The syntax of this function is
CSize GetTextEntent( LPCTSTR lpszString, int nCount ) const;
CSize GetTextExtent( const CString& str ) const;
Where
lpszString – Points to a string of characters.
nCount – Specifies the number of characters in the string.
str – A CString object that contains the specified characters.
Returns the dimensions of the string in a CSize object.
Example
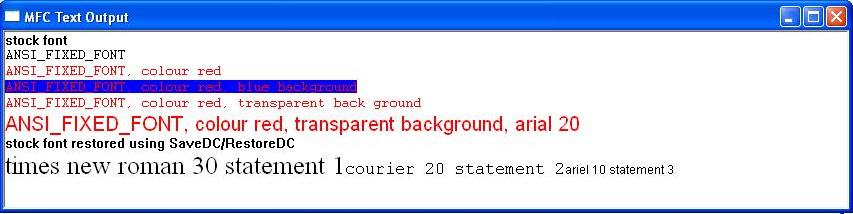
The following short program demonstrates the various text manipulation functions.
Download Code