The MFC Document View Architecture is a framework designed to separate the storage and maintenance of data from the display of data. This is achieved by encapsulating the data within a document class and the presentation specifics within a view class. The advantage of decoupling the view from the application data is of most use in larger applications when there are multiple views and multiple types of data objects to incorporate into an application.
MFC supports two types of document/view applications: single-document and multiple-document interface (SDI) applications.
Single Document interface
The expression Single Document Interface or SDI refers to a document that presents only one view to the user. This means the application is limited to displaying one document at a time. Notepad is an example of an SDI application. Notepad cannot open multiple text files without starting up another application instance. A simple single document/view application will contain four key class components-
The CDocumet class provides the basic functionality for an application’s document object. This functionality includes the ability to create load, save and update documents. This process of writing or reading data to a storage medium is known as serialization. The serialization objects supplied with MFC provide a standard and consistent interface, relieving the user from the need to perform file operations manually.
The CView Class encapsulates the document’s visual presentation rendering an image of the document on the screen or printer and handling user interaction through the view window. MFC provides several variations on the view class and the capabilities of a view class will depend on the MFC view class from which it derives. Further information about these view classes can be found at the following
https://docs.microsoft.com/en-us/cpp/mfc/derived-view-classes-available-in-mfc?view=vs-2019
The CMainFrame class encapsulates the frame window. MFC places the application view window into the client area of the frame window. In an SDI application, the view window is a child of the main frame window.
The CWinApp class is the base class from which every MFC programmer derives a Windows application object. This application object provides member functions for initialising and running the application.
Dynamic creation
In a document view application the view, frames and document objects are created dynamically using the following macros
DECLARE_DYNCREATE (class name) – found in the class declaration and allows dynamic creation of the class
IMPLEMENT_DYNCREATE(class-name, parent name) – found inside the class declaration. The class name is the name of the class being enabled and the parent class is the name of the MFC base class
Once the documents view and frame class have been created they can be used as parameters in a runtime class structure which is used as a parameter to create the document template using the class CsingleDocTemplate. The prototype of this class is –
CSingleDocTemplate( UINT nIDResource, CRuntimeClass* pDocClass, CRuntimeClass* pFrameClass, CRuntimeClass* pViewClass );
Where
nIDResource – Specifies the ID of the resources used with the document type.
pDocClass – Points to the CRuntimeClass object of the document class.
fFrameClass – Points to the CRuntimeClass object of the frame window class. This class can be a CFrameWnd-derived class, or the CFrameWnd itself.
pViewClass – Points to the CRuntimeClass object of the view class. This class is a CView-derived class you define to display your documents.
The newly created template can be added to the list of available document templates available to the application by calling the AddDocTemplate member function.
The CCommandLineInfo class encapsulates command line information when an application starts
And the ParseCommandLine parses the command line information.
Saving and loading documents
In a document view program the Serialize member function, defined in the CObject class, is responsible for loading or saving an object’s current state. The Serialize function contains a CArchive argument that is used to read and write the object data. The IsStoring or IsLoading member functions indicate whether Serialize is storing (writing data) or loading (reading data). An application either inserts or retrieves an application object’s data using the insertion operator (<<) or extracts data with the extraction operator (>>).
Example
In the following example, an SDI application is created which places a sequence of markers at the position of the mouse click. Selecting the relevant display option will change the display marker from x to asterisk and vice versa while the various file/save, file/load and /file/recent demonstrate how to save and load data using the serialize function
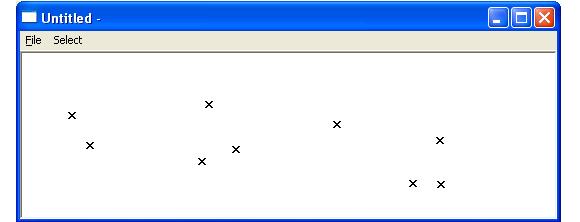