The following short program creates a listbox and then adds a limited number of selectable items. Clicking any list item results in the selected listview value being copied to the static box. Clicking button adds a new record, clicking the amend button amends the selected listview value and clicking the delete button deletes the selected listview item.
The Clist member functions AddString,DeleteString and GetString are used to set the add, delete and get the selected listbox content and the ON_LBN_SELCHANGE maps the combo box change event to the associated function
For further reading on the CListBox class
https://docs.microsoft.com/en-us/cpp/mfc/reference/clistbox-class?view=vs-2019
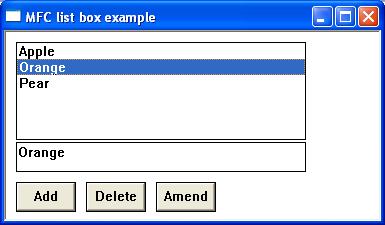
#include <afxwin.h> #define ID_ADDBUTTON 1000 #define ID_DELETEBUTTON 1001 #define ID_AMENDBUTTON 1002 #define ID_STATIC 1003 #define ID_EDIT 1004 #define IDC_LIST1 1005 class CSimpleApp : public CWinApp { public: BOOL InitInstance(); }; class CMainFrame : public CFrameWnd { public: CMainFrame(); afx_msg void wAddButtonOnClick(); afx_msg void wDeleteButtonOnClick(); afx_msg void wAmendButtonOnClick(); afx_msg void wListBoxChange(); DECLARE_MESSAGE_MAP() //instantiate button class; CButton wAddButton; CButton wDeleteButton; CButton wAmendButton; //instantiate edit class; CEdit wEdit; //instantiate listbox class; CListBox wListBox; }; BOOL CSimpleApp::InitInstance() { m_pMainWnd = new CMainFrame(); m_pMainWnd->ShowWindow(m_nCmdShow); return TRUE; } CMainFrame::CMainFrame() { Create(NULL,TEXT("MFC list box example"), WS_OVERLAPPEDWINDOW ,CRect(25,25,410,250)); wListBox.Create(WS_CHILD | WS_VISIBLE | LBS_STANDARD,CRect(10,10,300,110), this, IDC_LIST1); //add listbox records wListBox.AddString(TEXT("Apple")); wListBox.AddString(TEXT("Orange")); wListBox.AddString(TEXT("Pear")); wEdit.Create(WS_CHILD | WS_VISIBLE | WS_BORDER , CRect(10,110,300,140), this, ID_EDIT); wAddButton.Create(TEXT("Add"),BS_PUSHBUTTON | WS_CHILD | WS_VISIBLE | WS_BORDER, CRect(10,150,70,180), this, ID_ADDBUTTON); wDeleteButton.Create(TEXT("Delete"),BS_PUSHBUTTON |WS_CHILD | WS_VISIBLE | WS_BORDER , CRect(80,150,140,180), this, ID_DELETEBUTTON); wAmendButton.Create(TEXT("Amend"),BS_PUSHBUTTON | WS_CHILD | WS_VISIBLE | WS_BORDER , CRect(150,150,210,180), this, ID_AMENDBUTTON); } BEGIN_MESSAGE_MAP(CMainFrame,CFrameWnd) //message map ON_BN_CLICKED(ID_ADDBUTTON,wAddButtonOnClick) ON_BN_CLICKED(ID_DELETEBUTTON,wDeleteButtonOnClick) ON_BN_CLICKED(ID_AMENDBUTTON,wAmendButtonOnClick) ON_WM_PAINT() ON_LBN_SELCHANGE(IDC_LIST1,wListBoxChange) END_MESSAGE_MAP() CSimpleApp MFCApp1; //add item to listbox afx_msg void CMainFrame::wAddButtonOnClick() { CString strText; wEdit.GetWindowText(strText); if (strText!="")//check textbox is not empty { wListBox.AddString(strText); } } //delete selected item from listbox afx_msg void CMainFrame::wDeleteButtonOnClick() { int i = wListBox.GetCurSel(); if (i!=-1)//checks listbox item is selected { wListBox.DeleteString(i); wEdit.SetWindowText(""); } } //amend listbox item afx_msg void CMainFrame::wAmendButtonOnClick() { CString strText; wEdit.GetWindowText(strText); int i = wListBox.GetCurSel(); if (strText!="" && i!=-1)//checks textbox is not empty { wListBox.DeleteString(i); wListBox.AddString(strText); } } //detects for selected listbox change afx_msg void CMainFrame::wListBoxChange() { CString strText; int i = wListBox.GetCurSel(); wListBox.GetText(i, strText); wEdit.SetWindowText( strText);//sets textbox to selected listbox item }