The mapping mode governs how Windows translates logical coordinates into device coordinates within the current device context. Logical coordinates represent the graphics and text application values and the device coordinates are the resulting pixel positions within a window. The mapping mode also determines the orientation of the X-axis and the Y-axis and whether the values of X and Y increase or decrease with respect to the origin. The default device context sets logical units the same as pixels with the X axis being right positive, the Y axis being positive down, and sets the coordinate origin to the upper left corner of the window. Windows defines eight mapping modes. These are listed below
Mapping Mode | Logical Unit | x-axis and y-axis |
MM_TEXT | Pixel | Positive x is to the right; positive y is down |
MM_LOMETRIC | 0.1 mm | Positive x is to the right; positive y is up. |
MM_HIMETRIC | 0.01 mm | Positive x is to the right; positive y is up. |
MM_LOENGLISH | 0.01 in | Positive x is to the right; positive y is up. |
MM_HIENGLISH | 0.001 in | Positive x is to the right; positive y is up. |
MM_TWIPS | 1/1440 in | Positive x is to the right; positive y is up. |
MM_ISOTROPIC | user-specified | user-specified |
MM_ANISOTROPIC | user-specified | user-specified |
To select a different mapping mode, use the CDC member function SetMapMode() –
virtual int SetMapMode( int nMapMode );
where
nMapMode specifies the new mapping mode. The Return Value is the previous mapping mode.
Programmable Mapping Modes
The MM_ISOTROPIC and MM_ANISOTROPIC mapping modes differ from the other mapping modes in that the unit of measurement used to transform logical coordinates to device coordinates is user-defined. The MM_ISOTROPIC and MM_ANISOTROPIC mapping modes differ from each other in that with the former, the range of the x-axis and y-axis must be the same, and with the latter, the range of the x-axis and y-axis can be different. Selecting either of these modes means the developer will need to set the Window dimensions.
To set the logical extents of the window associated with the device context using the SetWindowExt() member function. To map the corresponding device size, known as the viewpoint onto these logical coordinates use the SetViewportExt() member function.
SetWindowExt
Sets the x- and y-extents of the window associated with the device context.
virtual CSize SetWindowExt( int cx, int cy );
virtual CSize SetWindowExt( SIZE size );
Parameters
cx – Specifies the Window x-extent (in logical units).
cy – Specifies the Window y-extent (in logical units).
size – Specifies the Windows x- and y-extents (in logical units).
Returns the previous extents of the window as a CSize object. If an error occurs, the x- and y-coordinates of the returned CSize object are set to 0.
SetViewportExt
Sets the x- and y-extents of the viewport of the device context.
virtual CSize SetViewportExt( int cx, int cy );
virtual CSize SetViewportExt( SIZE size );
Parameters
cx – Specifies the x-extent of the viewport (in device units).
cy – Specifies the y-extent of the viewport (in device units).
size – Specifies the x- and y-extents of the viewport (in device units).
Returns the previous extent of the viewport as a CSize object. When an error occurs, the x- and y-coordinates of the returned CSize object are set to 0.
For example, if SetViewportExt is called with parameters 100,50 and SetWindowExt is called with parameters 100,100 this will mean that each logical unit in the X direction will equate to 1 device unit and each logical unit in the y direction will equate to 1/2 a unit in the device coordinate.
Moving the Origin
By default, a device context’s origin, regardless of the mapping mode is in the upper left corner of the display. The device context origin can be changed by the CDC member functions SetWindowOrg() and SetViewportOrg() . The former moves the windows origin, and the latter, the viewport origin. The prototype for these functions are
CPoint SetWindowOrg( int x, int y );
CPoint SetWindowOrg( POINT point );
And
virtual CPoint SetViewportOrg( int x, int y );
virtual CPoint SetViewportOrg( POINT point );
where
cx – Specifies the x-extent (in logical units) of the window.
cy – Specifies the y-extent (in logical units) of the window.
size – Specifies the x- and y-extents (in logical units) of the window.
Returns the previous origin or viewport of the window as a CPoint object
Example
The following short program draws 5 squares under different mapping to illustrate the different display characteristics of each
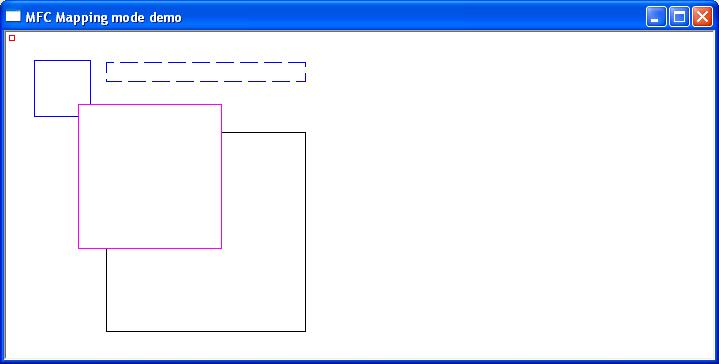